I am working using Fragments and I pull data from a MySQL database and put it into an ArrayList.
I then populate the Spinner with the returned data.
What I can't seem to figure out is how to customize that Spinner holding the data using the ArrayList I already have.
I define a spinner in my onCreateView as"
This is my class to extract the data, and how I am populating the Spinner:
This line populates the Spinner
The line right beneath it (commented out)
is the line that I can't quite figure out how to do it. The examples I have looked at show an Array being used, but I already have the ArrayList called trainers, so I don't know how to make use of it instead of creating another array.
Could someone assist me please?
Right now this is what my spinner looks like, very boring!
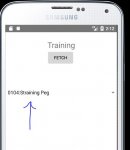
I then populate the Spinner with the returned data.
What I can't seem to figure out is how to customize that Spinner holding the data using the ArrayList I already have.
I define a spinner in my onCreateView as"
Code:
private Spinner spinner;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_training_layout, container, false);
spinner = (Spinner) view.findViewById(R.id.spinnerTrainers);
…
This is my class to extract the data, and how I am populating the Spinner:
Code:
private class ConnectMySql extends AsyncTask<String, Void, String> {
List<String> trainers = new ArrayList<String>();
ArrayAdapter<String> adapter;
@Override
protected void onPreExecute() {
super.onPreExecute();
}
@Override
protected String doInBackground(String... params) {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection(url, user, pass);
Statement st = con.createStatement();
ResultSet rs = st.executeQuery("SELECT * FROM pdc_production.die_detail");
ResultSetMetaData rsmd = rs.getMetaData();
while (rs.next()) {
trainers.add(rs.getString(1).toString() + ":" + rs.getString(2).toString());
}
} catch (Exception e) {
e.printStackTrace();
}
return "";
}
@Override
protected void onPostExecute(String result) {
adapter = new ArrayAdapter<String> (getContext(), android.R.layout.simple_spinner_dropdown_item, trainers);
// adapter = ArrayAdapter.createFromResource(getContext(), trainers, android.R.layout.simple_spinner_dropdown_item);
spinner.setAdapter(adapter);
}
}
This line populates the Spinner
Code:
adapter = new ArrayAdapter<String> (getContext(), android.R.layout.simple_spinner_dropdown_item, trainers)
Code:
adapter = ArrayAdapter.createFromResource(getContext(), trainers, android.R.layout.simple_spinner_dropdown_item)
Could someone assist me please?
Right now this is what my spinner looks like, very boring!
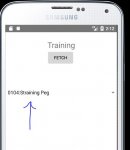